Mastering the C Programming Language: Finding Duplicate Characters in a String
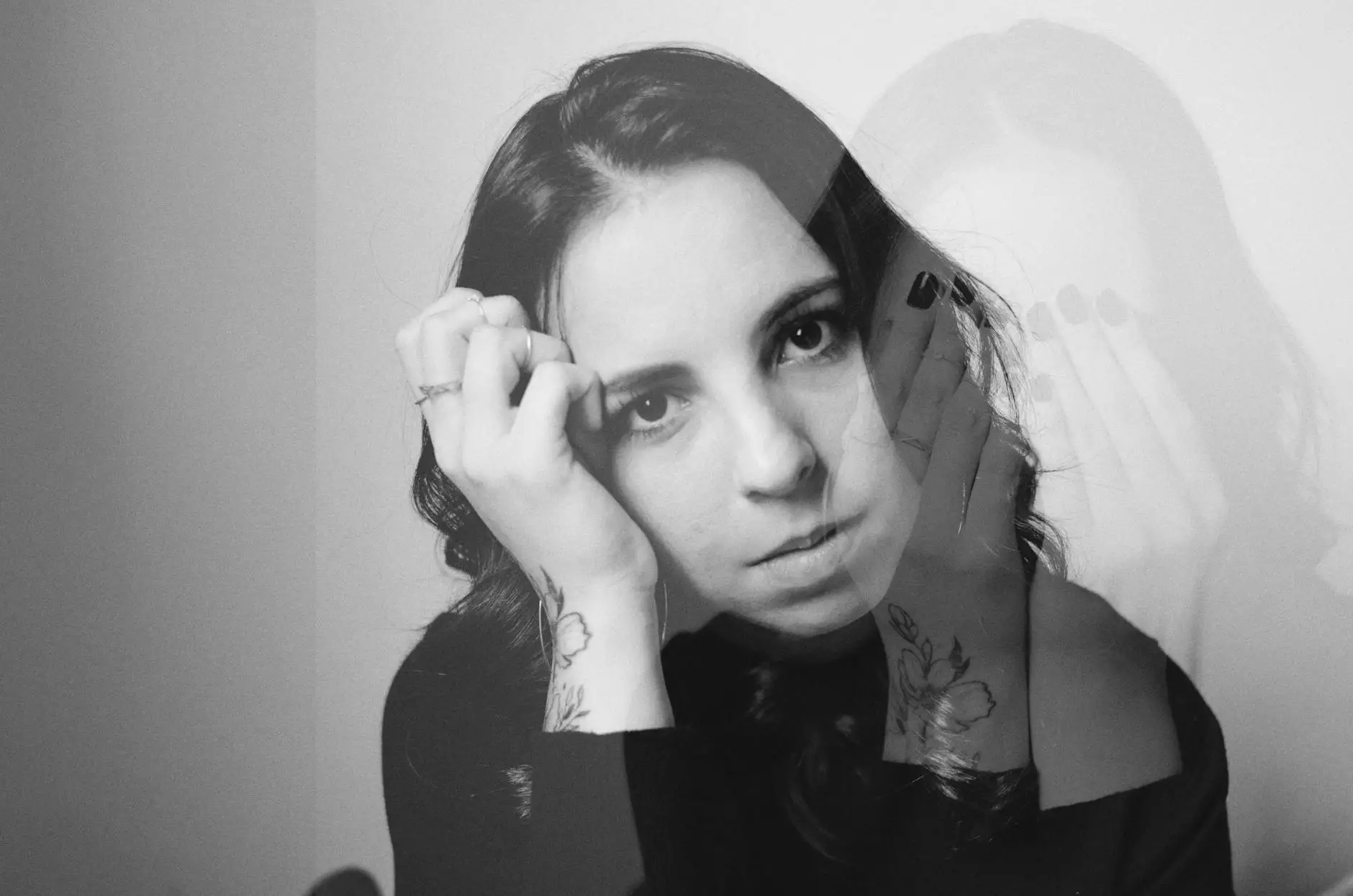
In the realm of programming, understanding how to manipulate and analyze strings is a fundamental skill. In this comprehensive guide, we will delve into the methods of identifying duplicate characters in a string in C. This article is designed for both beginners and experienced programmers who wish to enhance their C programming skills while learning to efficiently handle strings.
Understanding Strings in C Programming
Strings in C are fundamentally different from those in many other programming languages. In C, strings are basically arrays of characters terminated by a null character (\0). The importance of strings makes it essential for programmers to master various operations that can be performed on them. Here are some key points to remember:
- Strings are mutable: You can modify strings after declaring them.
- Memory manipulation: You have to manage memory explicitly in C.
- Character arrays: Strings in C are essentially arrays of characters.
Why Identify Duplicate Characters?
Before we throw ourselves into the code, let’s discuss the significance of identifying duplicate characters. This process can be essential in various scenarios such as:
- Data validation: Ensuring that specific inputs do not contain repeated characters.
- Compression algorithms: Removing duplicates can aid in compressing data.
- Text analysis: Analyzing text data for patterns or frequency of characters.
Setting Up the Environment
Before we begin coding, ensure you have the necessary environment set up to write and compile C code. You can use IDEs like Code::Blocks, Visual Studio, or even code in a simple text editor and compile using GCC. Make sure to have the C development environment ready to start coding your solution.
Basic Algorithm to Find Duplicate Characters
To find duplicate characters in a string, we can use a simple algorithm. The plan is to iterate through the string and keep track of the characters we’ve seen so far using an additional array. Here’s the step-by-step approach:
- Initialize an array to store the occurrence of each character.
- Loop through the string and check each character.
- If the character has been seen before, it is a duplicate.
- Store or print the duplicates as needed.
Implementing the Algorithm in C
Now let’s translate our algorithm into C code. Below is a simple program that demonstrates how to find duplicate characters in a string:
#include #include void findDuplicates(char str[]) { int count[256] = {0}; int i; // Loop through the string and count characters for (i = 0; str[i]; i++) { count[(int)str[i]]++; } printf("Duplicate characters in the string:\n"); for (i = 0; i < 256; i++) { if (count[i] > 1) { printf("%c occurs %d times\n", (char)i, count[i]); } } } int main() { char str[100]; printf("Enter a string: "); gets(str); findDuplicates(str); return 0; }Breakdown of the Code
Let's examine the code in greater detail:
- Header Files: We include stdio.h for input-output functions and string.h for string manipulation functions.
- Count Array: An integer array count[256] is used to record the occurrence of each character. The size of 256 accounts for all possible ASCII characters.
- Counting Logic: As we loop through the input string, we increment the count for each character based on its ASCII value.
- Printing Duplicates: Finally, another loop checks our count array, and if any character has a count greater than one, it prints that character along with its occurrence.
Optimizing Our Approach
While the above method is effective, it can be improved in terms of efficiency and space complexity. For smaller strings or specific character sets (like lowercase letters), we could reduce the space usage significantly. Here’s how:
#include void optimizedFindDuplicates(char str[]) { int count[26] = {0}; // For lowercase letters only int i; for (i = 0; str[i]; i++) { if (str[i] >= 'a' && str[i] 1) { printf("%c occurs %d times\n", i + 'a', count[i]); } } } int main() { char str[100]; printf("Enter a string: "); gets(str); optimizedFindDuplicates(str); return 0; }Advanced Techniques: Using Data Structures
In more advanced scenarios, you might want to utilize data structures such as hash tables or linked lists to manage duplicates, especially when dealing with larger data sets where performance is critical. For instance, you could use a hash table to store characters and their counts effectively, thereby reducing the traversal time.
Common Pitfalls to Avoid
When working with strings and duplicate character detection in C, keep the following common pitfalls in mind:
- Buffer Overflows: Always ensure that you do not exceed the bounds of your arrays.
- Ignoring Case Sensitivity: Decide if your character checks should be case-sensitive or case-insensitive and implement accordingly.
- Input Handling: Ensure proper handling of user input to avoid unexpected behavior.
Practical Applications of Duplicate Character Detection
The ability to find duplicate characters in strings has numerous real-world applications, including:
- Text Processing: For applications like spell checkers or word processors.
- Data Validation: In forms where unique usernames or identifiers are required.
- Compressing Data: By identifying and removing duplicates before encoding text data.
Conclusion
In conclusion, the ability to identify duplicate characters in a string in C is a vital skill for both beginner and advanced programmers. By employing various algorithms and techniques, you can efficiently solve the problem of duplicate detection in strings. This skill not only aids in programming exercises but can also provide significant benefits in practical applications.
As you continue your journey with C programming, remember that mastering string operations like this will give you a strong foundation for handling more complex data manipulation challenges in the future. Happy coding!
duplicate characters in a string in c 0934225077